In Part I, we talked about mocking some of our functionality using pgxmock
. In part 2, we will replace the manual approach with mockery
, a tool that automates the generation of mocks. It maintains the same structure and intent as the original article while focusing on the benefits and usage of mockery
.
Why Mock the Database?
When writing unit tests, we want to test the logic of our code in isolation. This means we should avoid dependencies on external systems like databases. By mocking the database, we can simulate different scenarios (e.g., successful queries, errors, etc.) without needing a real database connection.
Introducing Mockery
Mockery
is a popular Go tool that generates mocks for interfaces. It automates the process of creating mock implementations, saving us time and ensuring consistency in our tests. With mockery
, we can easily generate mocks for our database interfaces and use them in our unit tests.
Example: Mocking a Database Interface
Let’s walk through an example of how we use mockery
to mock a database interface in our unit tests.
Step 1: Define the Database Interface
We can reuse the same interface from part I:
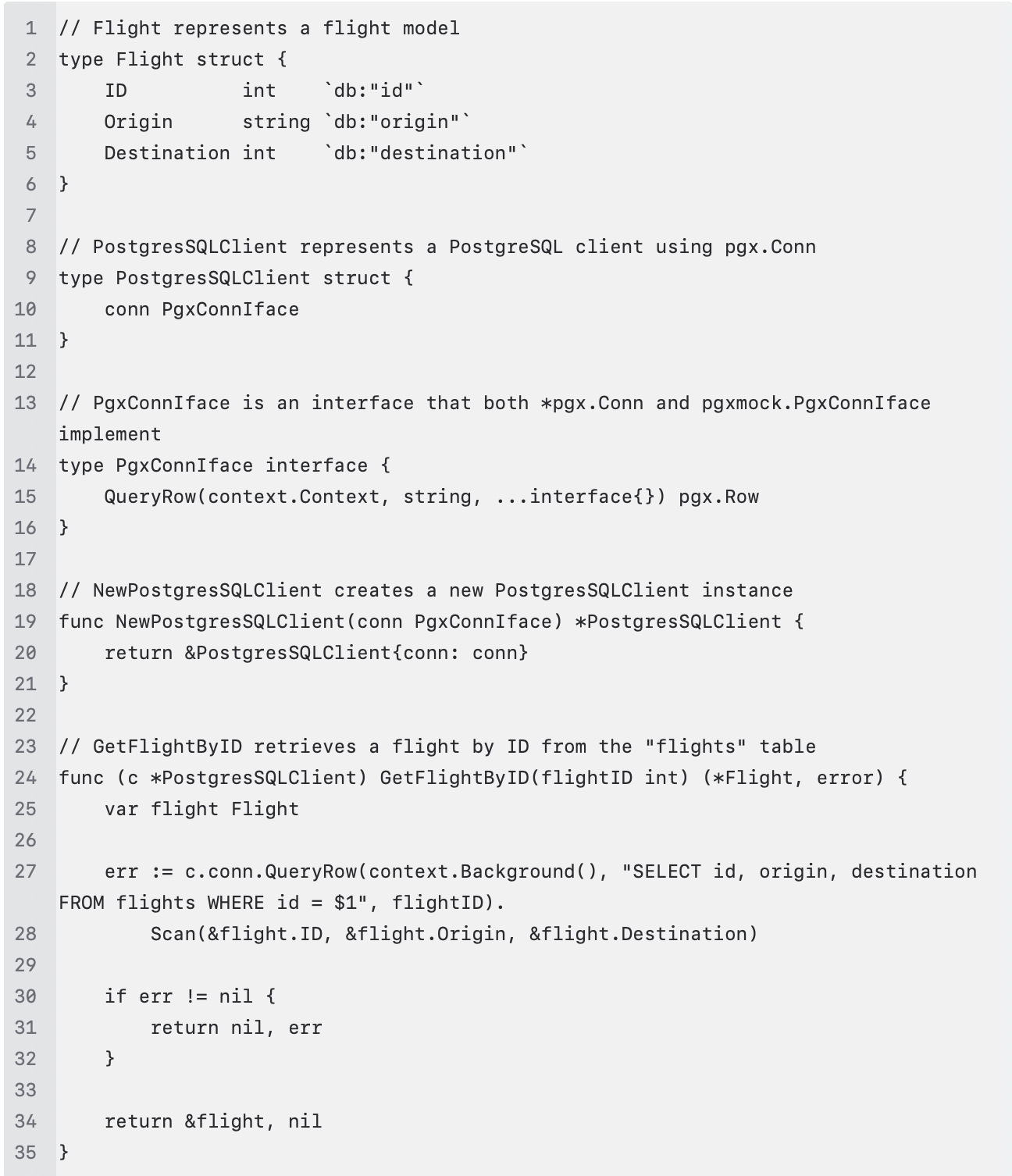
Step 2: Generate Mocks with Mockery
To generate a mock for the PgxConnIface
interface, we use the mockery
command-line tool. First, install mockery
if you haven’t already:
go install github.com/vektra/mockery/v2@latest
Next, run mockery
to generate the mock:bash
mockery --name=PgxConnIface --output=./mocks --outpkg=mocks --filename=pgx_conn_mock.go
This command generates a mock implementation of the PgxConnIface
interface in the mocks
directory. The generated file will be named pgx_conn_mock.go
and will look something like this:
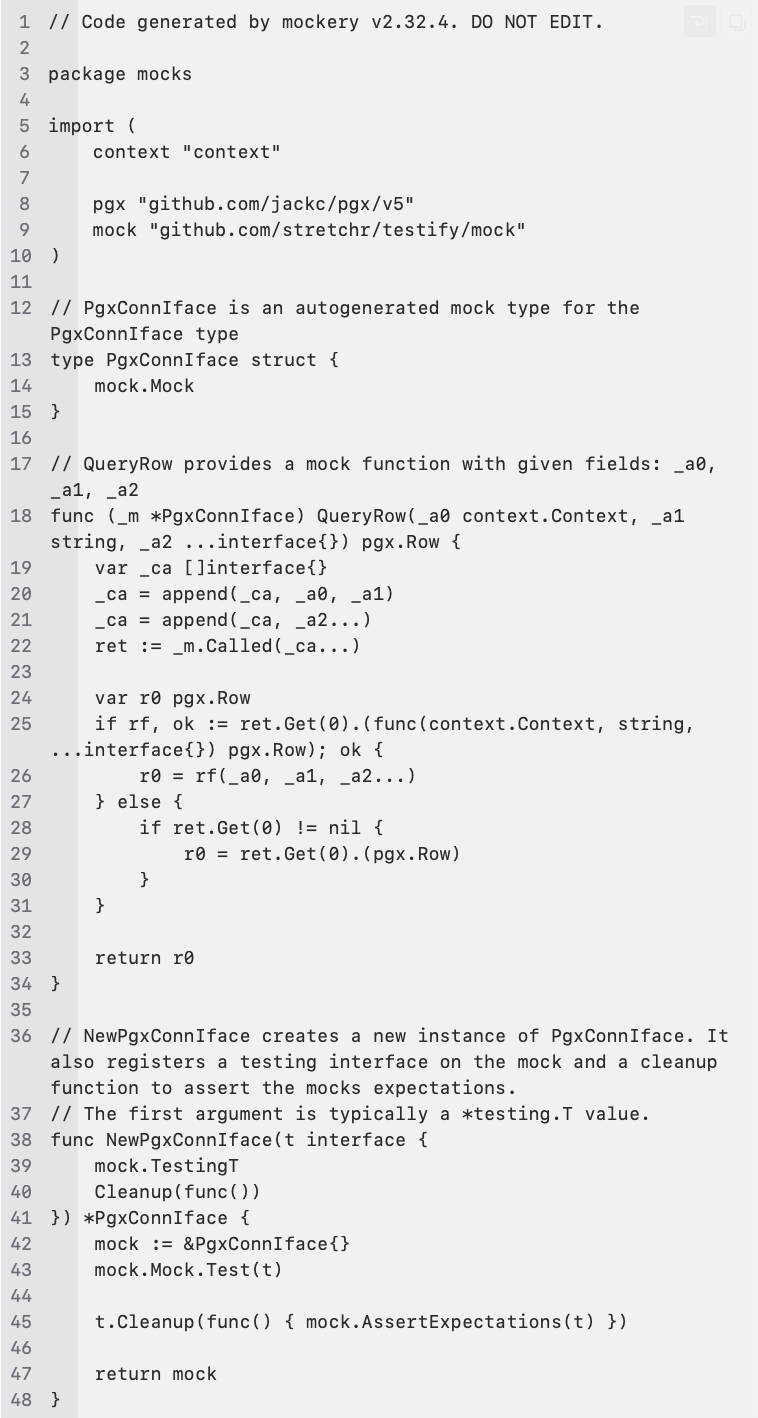
Step 3: Write Unit Tests Using the Mock
Now that we have a mock implementation of PgxConnIface
, we can use it in our unit tests. Here’s an example of how we might test functionality that depends on PgxConnIface
:
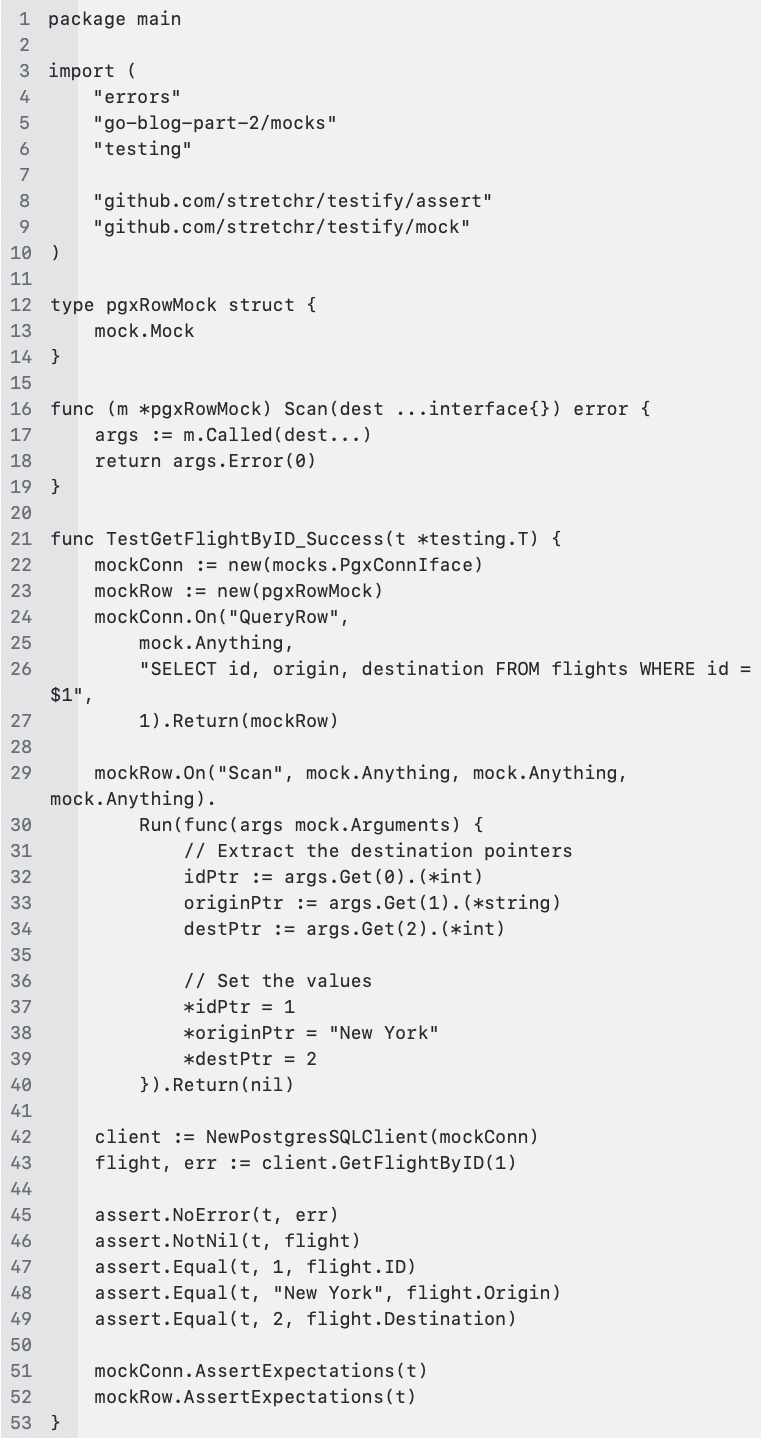
In this test:
- We create a new mock
PgxConnIface
using the generatedmocks.PgxConnIface
struct. - We set up expectations for the
GetFlightByID
method usingmockRepo.On
. - We call the method under test (
GetFlightByID
) and assert the results. - Finally, we verify that the mocks were called as expected using
mockConn.AssertExpectations
andmockRow.AssertExpectations(t)
.
Step 4: Test Edge Cases
One of the benefits of mocking is the ability to easily test edge cases. For example, we can simulate a database error to ensure our code handles it gracefully:
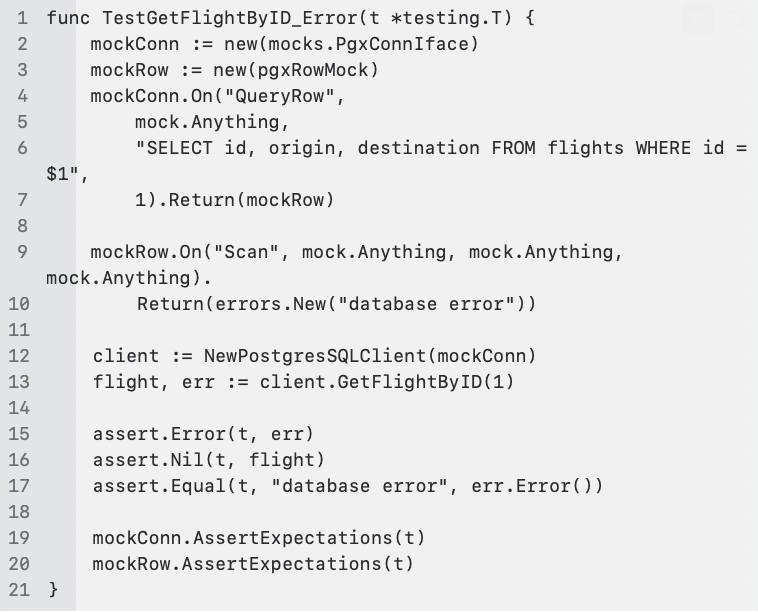
Conclusion
Using mockery
to generate mocks for our database interfaces streamlines the unit testing process. It allows us to easily simulate different database scenarios and ensures our tests are isolated from external dependencies. By following the steps outlined in this article, you can start using mockery
in your own Go projects to write more reliable and maintainable unit tests.
Happy testing!